This Plugin for Godot 3.x allows you to integrate your game with the Game Jolt API.
Installation
Unzip the file
gamejolt-api-plugin-v1.3.zip
in the root path of your game project.To activate the plugin, go to
Project > Project Settings > Plugins
, and change its status to Active .
Once installed, the plugin will automatically configure and load the GameJoltAPI singleton.
Now you can make API requests by calling the GameJoltAPI's functions.
Configuration
Use the following function to set up the Game API settings:
GameJoltAPI.set_game_credentials({
"game_id": "YOUR GAME ID",
"private_key": "YOUR PRIVATE KEY"
})
This function only needs to be called once, it is recommended to place it in the main scene of your game.
Note: You must call this function before making a request.
You can find the game credentials in Game Jolt, by going to the Game Page > Manage Game > Game API > API Settings.
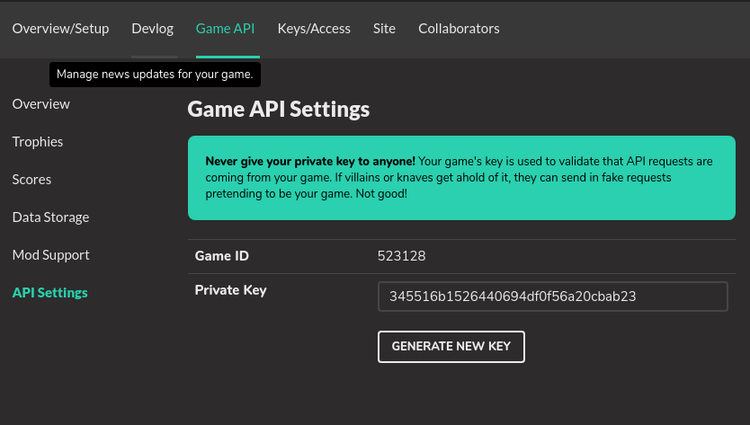
Getting the player's credentials
In order to automatically get the player's credentials in your game, you need to call the following function:
GameJoltAPI.get_user_credentials()
Note: This feature is only available when running the game in the Game Jolt Desktop App and Web builds.
You can manually set the player's credentials within the game (useful for debugging your game):
GameJoltAPI.username = "GAMEJOLT USERNAME WITHOUT @"
GameJoltAPI.user_token = "PLAYER'S GAME TOKEN"
Make your first GAME JOLT API request
Once you have set up the API, you are ready to make API requests.
Let's create a trophy!
You can add a new trophy by going to Game Page > Manage
Game > Game API > Trophies
. Introduce the Title
and add an Image
.
A Trohpy ID
is generated! , we will use it in our API request.
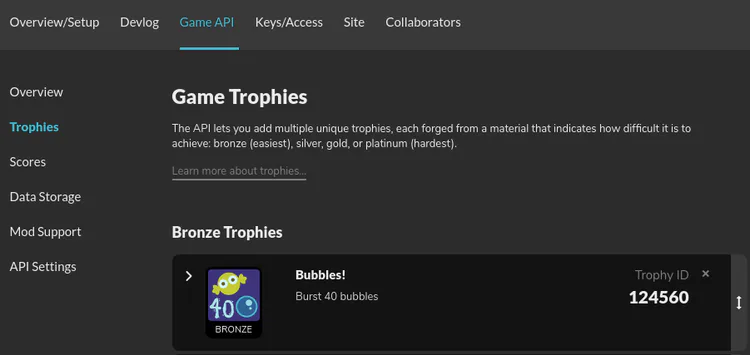
Let's make a request
Let's make the player achieve the trophy within the game by calling the following function:
var trophy_request = GameJoltAPI.add_achieved({
"username": GameJoltAPI.username,
"user_token": GameJoltAPI.user_token,
"trophy_id": "124560"
})
Note that we are using all the required parameters (except the game_id
) to make the API request.
For more information about the Game Jolt API - Trophies check the official Game Jolt API documentation.
Handle the API response
The GameJoltAPI requests have the following signals:
api_request_completed: This signal will be triggered when the request is successfully processed by the Game Jolt Server. This signal will return an Array containing the server response.
api_request_failed: This signal will be triggered if an error occurs during the sending or processing stage of the request. This signal will return an error message.
These signals can be connected via GDScript:
trophy_request.connect("api_request_completed",
self, "_on_request_completed")
trophy_request.connect("api_request_failed",
self, "_on_request_failed")
The following functions will be executed when the signals are triggered :
func _on_request_completed(data: Array) -> void:
#Do something...
pass
func _on_request_failed(error: String) -> void:
#Do something...
pass
For more information about the GamejoltAPI functions, check the GameJoltAPI Documentation.
Make a custom API request
In addition to the available GameJoltAPI functions, you can make your own custom API requests according to the Official Game Jolt API documentation:
In the following example, we saved a key-value pair in the GJ cloud-based data storage system:
var custom_request = GameJoltAPI.create_request(
"/data-store/set/",{
"key": "myData",
"data: "Hello World!"
}
#Connect to the signals:
custom_request.connect("api_request_completed", self, "_on_custom_request_completed")
Note: you must introduce all the required API parameters (except the "game_id").
Handle multiple API requests
The handle_requests
function allows you to trigger an action when all requests have been successfully completed. If one of the requests fails, you can trigger another action instead (this is optional).
In the following example, we want to display a message to the player once his score has been registered and he has won a trophy:
#request 1 (Saving the player score):
var score_request = GameJoltAPI.add_score({
"username": GameJoltAPI.username,
"user_token": GameJoltAPI.user_token,
"score": player_score,
"sort": player_score
})
#request 2 (Giving a trophy to the player):
var trophy_request = GameJoltAPI.add_achieved({
"username": GameJoltAPI.username,
"user_token": GameJoltAPI.user_token
})
#Handle both API requests:
GameJoltAPI.handle_requests(
[score_request, trophy_request],
self,
"_on_requests_completed",
"_on_requests_failed"
)
If both requests were successful, the function _on_requests_completed
will be called, returning as a parameter an Array with the responses given by the API.
Otherwise, if an error occurs in one of the requests, the function _on_requests_failed
will be called, returning the error message as a parameter.
func _on_requests_completed(responses: Array) -> void:
#Do an action...
pass
func _on_requests_failed(responses: Array) -> void:
#Do an action...
pass
Exporting your game
In order to perform API requests through the HTTPS protocol, it is necessary to use an SSL certificate. The plugin has the file ca-certificates.crt, which is automatically included in your project when you install the plugin.
Remember to add .crt into the filters so the exporter recognizes this when exporting your project.
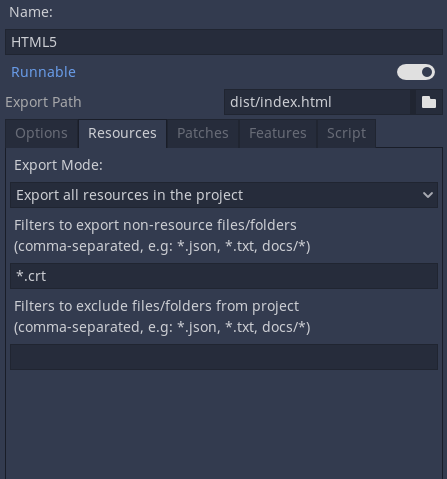
And that's it! If you want more information about the available functions, check out the GameJoltAPI Documentation.
12 comments