Comments
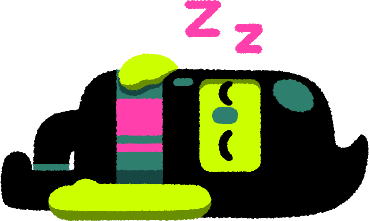
No comments yet.
Free
NIL Isn't Lua
You can download the current version here, BUT, if you want to make sure you always have the latest version, you can best clone or download from my github repository: https://github.com/jpbubble/NIL-isn-t-Lua
Since NIL is written in pure Lua code, it's platform independent. As long as Lua engines (Lua version 5.1 or later) exist for the OS you want to use it on, NIL should work in it
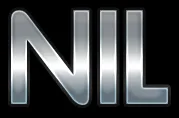
NIL is a kind of programming language created in Lua, allowing several enhancements to Lua. Any engine using Lua 5.1 or higher should be able to support NIL.
The easiest way to make your engine use NIL is by copying NIL.lua into your project directory where your main Lua file lives and type the next two lines:
NIL = require "NIL"
NIL.Use("MyNilProject.nil")
What is the advantage of using NIL in stead of Lua?
Lua is a very quick and lightweight scripting language, and it's simplicity is its power and weakness. When using Lua for quick configuration settings or very small addons (for which it was originally intended), Lua can work best, as those kinds of things require a quick way of coding and Lua has a very quick syntax. When you're going to use Lua for long complex serious projects, then it can become bothersome. One misspelled identifying name can lead to bugs that can take forever to fix, and since Lua was never really set up for OOP programming, this can be quite a chore. Don't get me wrong OOP is possible in Lua, but not officially supported, you can just "cheat your way around a little", but this can get messy in the longer run, though.
Therefore NIL require all identifiers to be declared. Just like in C
string name = "Jeroen"
int year = 1975
int thisyear = 2019
print(string.format("I am %s and I was born in %d and thus %d years old",name,year,thisyear-year))
As you can see, NIL can just pick up the stuff Lua provides automatically, so print and string.format will just work the same way. The declaration is inspired on how it works in C.
Like I said, Lua has no official OOP support. There are ways to "cheat" around this, but it's not very cool. NIL still allows you to follow these "cheats", no problem there, but why go down that way if you can make NIL do this for you:
class Person
static int year=2019
string name
int yob
void CONSTRUCTOR(n,y)
self.name=n
self.yob=y
end
get int age
return Person.year - self.yob
end
end
var me
me = Person.NEW("Jeroen",1975)
print(string.format("I am %s and I am %d years old!",me.name,me.age))
switch statements were never supported in Lua. NIL *does* support them.
string animal = "Dog"
switch animal
case "Dog"
print("Woof")
case "Cat"
print("Meow")
case "Cow"
print("Moo")
default
print("I don't know THAT animal")
end
Lua does not support constants. Now NIL has no official support for constants either, but it does support macros in stead
#macro JustMyName Jeroen
#macro StrMyName "Jeroen"
print("JustMyName")
print(StrMyName)
It is very important you understand that macros are not identifiers. NIL will just replace them with the value you set for them, similar to how macros work in C and C++, however the feature is less sophisticated than their C/C++ variants, they are still pretty useful. You can prevent "magic values" without risking stuff to be overwritten.
NIL and Lua can exchange stuff with each other. And basically except for Macros which can not live in Lua (sorry) you can basically exchange anything. Yes, even classes defined in NIL.
MyClass.nil
class MyClass
string n
int age
end
MyTest.lua
NIL = require("NIL.lua")
NIL.Use("MyClass.nil")
MC = MyClass.NEW()
MC.name = "Jeroen"
MC.age = 44
But the other way around will also work
MyTest.nil
#use MyFunction
Hello()
MyFunction.lua
function Hello()
print("Hello World")
end
What does NIL actually do? NIL just translates all nil-scripts into pure Lua code (one little cheat on the classes for which I added a meta-table, which is just a Lua standard feature), and Lua will just run the translated code like it were normal Lua code. The translator tries to keep the line numbers the same, in order to make run-time errors display the same numbers as if the code was written in pure Lua.
Is it as fast as Lua? What goes for all trans-compilers is "if you want to pure power of the target language use that target language". When you use a language translating to C, then C will always be faster than the language you used to translate to C, and C can never be as fast as coding directly into machine language. NIL is no different on that department, however NIL has been designed to keep its syntax as close as possible to Lua, and on many occasions NIL only checks if the used keywords and identifiers exist and are properly used and will leave the rest to Lua itself. Loading NIL scripts can take a bit longer, since NIL first needs to be translated to Lua, during run-time, in most cases the difference should be hardly noticeable... if there is any difference at all.
Is NIL better than Lua? That depends on YOUR definition of the word "better". For me it is better in larger projects. If you don't like these features, then maybe NIL isn't for you. Remember, the best programming language does not exist.
How can Lua use NIL classes? The trick lies that NIL has a few meta-tables completely set up for the usage of classes. Meta-tables are however a very awkward beast to deal with, and although they can provide a lot of functionality, having to set them up for every class variable can be a terrible experience. NIL just takes that out of your hands. However since NIL uses functionality that just comes with Lua by default, Lua won't recognize it as a NIL translation, but just a valid code it can run. And that's how you can just uses classes created with NIL even in pure Lua code.
Can NIL still used "in-lined" Lua code? Yes, it can! However this is pretty risky, and should only be used if you know what you are doing. Read the wiki page to see full information about how this works, and what risks you take this way.
If you have any issues in NIL please go to my issue tracker and report them to me.
#programminglanguage #nil #lua #easy #easytouse #easytolearn #translator