For my upcoming games I’ve created my own “programming language” named NIL. Of course, I already have the Scyndi programming language, but NIL was set up from a completely different perspective than Scyndi, so it would be best to even TRY to compare the two.
NIL is an acronym and stands for “NIL isn’t Lua”. Well, I guess that makes the relation with Lua a bit clear, but that it is slightly different. NIL is a kind of pre-processor for Lua, written completely in Lua.
This concept is not entirely new. As there’s also MoonScript which is also a pre-processor for Lua written in Lua, but MoonScript is in my humble opinion completely beyond all levels of being terrible, and beyond human understanding, and even viewing the simplest code written in it cripples the mind and teaching it should therefore be regarded as a criminal offense, especially since Lua was meant to be easy, and MoonScript defies that very objective. For JavaScript similar project exist such as TypeScript and CoffeeScript. NIL is my addition to this family of languages.
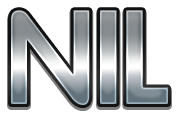
Now why did I create NIL? Wasn’t Lua easy enough? Well, Lua’s simplicity is as well a blessing as it is a curse. Lua lacks a few important things to make coding in it a real joy, and where Lua also can give you headaches is the fact that Lua does not require to declare your identifiers and will regard any undefined identifier as a “nil-value” (perhaps the name of this language is also a bit a pun on that pain). I’ve written games in Lua with over 50,000 lines of Lua code, and if one identifier contains a typo, you’re in for a very rough ride to find out in which line the identifier has been misspelled. In small addons written in Lua (for which Lua was actually intended… figures), this won’t matter, but in games with over 50,000 lines of Lua code, this is the road to Hell!
The objective of NIL was to stay as close to the Lua syntax as possible, but to change/add that some of the pains Lua has can be avoided. NIL does therefore require variable declaration (to protect you against typos), but it does more. It also features a robust class support (which Lua doesn’t support at all), a “switch” statement (why didn’t Lua have this from the start), a macro system in order to get some support for constants (variables are too dangerous for this, as they can be modified, however as you’d otherwise end up with “magic numbers” which is one of the biggest sins a coder can commit, especially in big projects) and a few other enhancements.
All you need is the file NIL.lua and to put this line on top of your code:
NIL = require "NIL"
Now write your program in NIL and you can just import it into Lua like this:
myprog = NIL.Use("MyProgram.nil")
NIL has been designed to be operate as seamlessly with Lua as possible. Any Lua global that was not ‘nil’ prior to loading a NIL-script will be recognized by NIL and you can just use it in NIL just as it were written in NIL, but also vice versa. Yes, even classes declared in NIL can be used in Lua the same way as you’d use them in NIL. This is where NIL shows its power.
Let’s not break with traditions…
void HW()
print("Hello World!")
end
HW()
As you can see the only difference above is that “function” has been replaced with “void” to deterime that the function shouldn’t return a value (just like how that works in C and most of its variants).
Let’s put in something more complex, with classes and the switch statement
#macro YearNow 2019
class ClassMates
string Name
int YearOfBirth
int Gender
get int Age
return YearNow - self.YearOfBirth
end
get string GenderName
switch self.Gender
case 1
return "Male"
case 2
return "Female"
default
error("Unknown gender code!")
end
end
void CONSTRUCTOR(name,YOB,Gender)
self.Name=name
self.YearOfBirth
self.Gender = Gender
end
end
var CM
CM = ClassMates.NEW("Jeroen",1975,1)
print(CM.Name.." is a "..CM.Age.." years old ".. CM.GenderName)
I’ve also provided a syntax highlight script for the Geany editor ;)
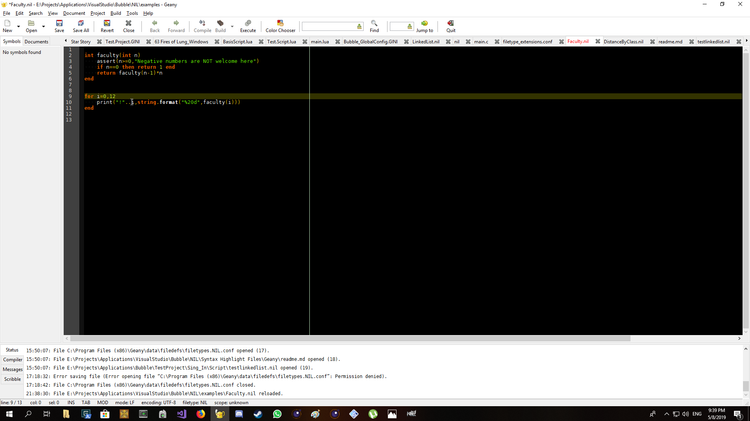
(Magnification can be found here: https://github.com/jpbubble/NIL-isn-t-Lua/blob/master/Syntax%20Highlight%20Files/Geany/Screenshot%20in%20Geany.png )
NIL is not perfect yet, but it is already usable and it should work on any engine using Lua 5.1 or later.
You can find out about NIL on my GitHub respository, where there’s also a wiki going more into the deep of how NIL works. I hope you like it… I am already planning to use it in my future projects, and maybe even after Cynthia Johnson has been completed (For Cynthia Johnson it’s really too late to add NIL-scripts now).
11 comments