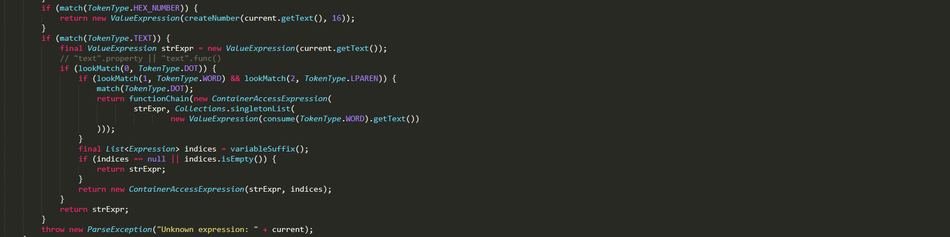
Comments (1)
выглядит как проект который я бы не осилил
Elixir
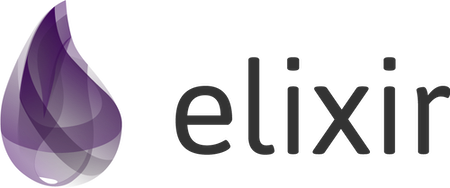
Elixir - interpreted dynamic functional programming language inspired by Scala and Python. Available for PC.
REQUIERS JAVA RUNTIME ENVIROMENT!
The project is currently under development.
Full source code available here.
Key Features
Ternary Operator
printf "Ternary operator"
a = 0
b = 1
printf (a ? "text1" : "text2")
printf (b ? "text3" : "text4")
Extended identifier variables
`extended identifier variable` = 9
printf (`extended identifier variable`)
`(。◕‿◕。)` = 20
`ʕ•ᴥ•ʔ` = 30
printf(`(。◕‿◕。)` * `ʕ•ᴥ•ʔ`)
Maps
map1 = {"key": "value"}
printf(map1["key"])
printf(map1.key)
map1["newkey"] = "newvalue"
map1[0] = "zero"
x = 400
map1[x] = [1, 2, 3]
printf(map1)
Syntax sugar ( '=' instead of reuturn operator )
def sqrt(num) = num*num
for (i = 0, i < 6, i++) {
printf("15 in square = " + sqrt(15))
}
Why Elixir ?
Elixir is written in Java which provides stability and high speed of work
Simple, no-nonsense syntax
Fast, and lightweight Interactive Shell !
Basics
Comments
// Line comment
/* multiline
comment
*/
printf /*inner comment*/ "Text"
Strings
Strings are defined in double quotes and can be multiline. Escaping Unicode characters is also supported.:
str = "\n\tThis is
\tmultiline
\ttext
"
printf(str) //print statement
printf
and printn
operators are used to output text.
Types
Elixir types are:
Number - numbers (integer, float)
String - strings
Array - arrays
Map - objects (an associative arrays)
Function - functions
Since Elixir is dynamic programming language, which means that explicitly declare the types is not necessary
vx = 10 // integer
y = 1.61803 // float
z = "abcd" // string
If some function requires string as argument, but number was passed, then numeric value will automatically converts to string.
x = 90
printf(x) // Ok, 90 converts to "90"
Loops
Example :
while condition {
body
}
Parentheses in condition are not necessary.
i = 0
while i < 5 {
printf(i++)
}
// or
i = 0
while (i < 5) {
printf(i++)
}
Do-While Loop soon.
For Loop.
for initializing, condition, increment {
body
}
for (initializing, condition, increment) {
body
}
for i = 0, i < 5, i++
printf(i++)
// or
for (i = 0, i < 5, i++) {
printf(i++)
}
Functions definition
To define function uses the def
keyword:
def function(arg1, arg2) {
arg1++
}
Shorthand definition
There is short syntax fot function body:
def repeat(str, count) = str * count
Which is equivalent to:
def repeat(str, count) {
return str * count
}
Default arguments
Function arguments can have default values.
def repeat(str, count = 5) = str * count
In this case only str
argument is required.
repeat("*") // *****
repeat("+", 3)
Default arguments can't be declared before required arguments.
def repeat(str = "*", count) = str * count
Causes parsing error: ParseError on line 1: Required argument cannot be after optional
Inner functions
You can define function in other function.
def fibonacci(count) {
def fib(n) {
if n < 2 return n
return fib(n-2) + fib(n-1)
}
return fib(count)
}
printf(fibonacci(10)) // 55
Assertion
Example:
def check(a,b) {
assert(a + b == 0)
}
check(15,8)
Terminate Program
Example:
def say() {
printf("Hi!")
terminate //program will be closed
printf("Goodbye!") // It's invisible
}
say()