Are globals evil?
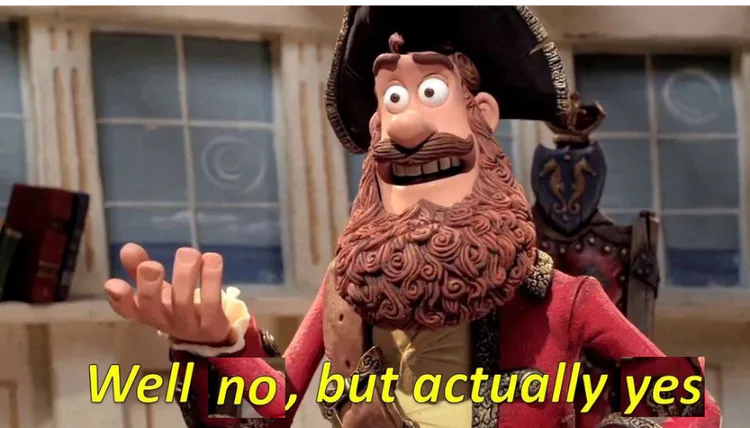
It is always a debate. I'd say they are not evil. Use them if you have to, but don't overuse them. Globals do have an important purpose in coding.
Globals are considered a no-no by many coders, along with the jump-instruction (aka "goto") and the if-instruction. Where the goto instruction easily causes nothing but incomprehensible chaos, and the use of "if" could sometimes be voided depending on the situation, globals do have their pros and their cons.
Well, the basic rule I uphold in my own coding work is. Avoid them if you can, use them if you must. Unlike "goto" some code has been turned into chaos beyond insanity all due to the desperate desire to never use globals and if-statements, and I often wonder if that is worth it.
Let's get to the point. for those pretty new to coding a short explanation on what globals are. A global is a variable that is available in the entire scope of your code, a local is only available within the scope of your program in which it is declared/defined.
Let's give a little demonstration in C++
#include <iostream>
using namespace std;
int myglobalvar = 0;
void mydefine() {
myglobalvar = 125;
int mylocalvar = 20;
}
int main() {
mydefine();
cout << myglobalvar << "\n";
cout << mylocalvar << "\n";
}
This code will cause an error when you try to compile it. Trust me. It will say that "mylocalvar" is unknown. And that is logical. mylocalvar is a local variable declared in the mydefine() function, yet I tried to call it in the main() function, and that doesn't work. myglobalvar however is a global variable, so that works fine. Should I move the "cout << mylocalvar" line to the mydefine() function like below, it will work:
#include <iostream>
using namespace std;
int myglobalvar = 0;
void mydefine() {
myglobalvar = 125;
int mylocalvar = 20;
cout << mylocalvar << "\n";
}
int main() {
mydefine();
cout << myglobalvar << "\n";
}
Now to the question my globals are "evil". That is easy to explain. Globals can be defined over the entire scope of your program, that's why. Every function, every portion of your code has access to them. You do not want that. It can easily cause bugs when you lose track of what has access to a variable and what has not. By limiting the access to variables as much as possible, especially when it comes to altering them, you can prevent a lot of trouble.
A C++ joke says "What is the best way to prefix a global? Answer //"
Well prefixing globals *can* be a good idea, so you can be sure you can easily distinguish globals from locals in order to prevent real trouble.
Of course, another reason to prevent globals for is that in coding there are tons of data you only need for a short amount of time, and after that never again. Particularly in complex mathematical calculations, and most notably iteration variables in for-loops. Although C, C++, Pascal, BASIC and loads of other languages do allow the usage of globals for for-loops, the practice is strongly discouraged. Personally, I wouldn't go there. Some other languages (like Lua for example) automatically declare a local that only works inside the for-scope, which is something I personally only applaud. Not only can using locals for this cause trouble in creating chaos (particularly when using recursive function calls), they only eat RAM needlessly as well. I mean every variable you use, needs to be allocated. This normally happens automatically (yes, even in C. C only needs manual allocations and releases when you work with pointers, but I am not talking about those). When using a global for a for-loop or for data only a certain function needs, the memory will always be taken up. Do we need that? Nope. So when using a local we know at least the memory it took will be used elsewhere when the scope it was used in has been left.
Now I do need you to watch out. An overuse of locals can require multiple functions to have the same data and well, take up extra RAM needlessly too. Locals do not solve all issues... They can sometimes even create them.
Static locals
So far, I've only found TWO languages out there supporting this (not counting the languages I set up myself). That would be C and C++. It's possible there are other languages out there of which I don't know it. C# does NOT seem to support it, and it's beyond me why.
For RAM saving statics are not the way, but for preventing bugs caused by unauthorized access to globals, this will definitely save you a lot of headaches.
I'll write the same program in C twice. With and without a static local.
// Without static
#include <stdio.h>
int counter = 0;
void count(void) {
counter++;
printf("%3d\n",counter);
}
int main(void) {
count();
count();
count();
count();
count();
count();
count();
count();
count();
count();
count();
return 0;
}
You see, this sucks.... the "counter" variable is a global now, but what for? The "count()" function is the only function using it, yet main() has access to it... for no reason. Yes, this can prevent bugs, so if you are using a language that supports static locals.... USE THOSE IN STEAD!!!
// Without static
#include <stdio.h>
void count(void) {
static int counter = 0;
counter++;
printf("%3d\n",counter);
}
int main(void) {
count();
count();
count();
count();
count();
count();
count();
count();
count();
count();
count();
return 0;
}
Now, we're talking. For starters the counter=0 definition is only execution the first time the count() function is called. After that the value always stays the same UNLESS other instructions (in this case counter++) alter it. The output this program generates is exactly the same. This time the main() function (nor any other function except count() for that matter) has action to the counter variable. Although whenever count needs it, it can still access this. Since globals are hated so much, I do think it's odd so little programming language appear to have support for this.
Why didn't I use a for-loop above? Because it would kill what I was trying to demonstrate. That code is a TUTORIAL, not a serious software solution! It's sad I have to write this disclaimer, but unfortunately, some people are idiots who think they can hide it by making "clever" remarks.... sigh! I consider them as trolls, and they will be dealt with accordingly (read: comments like that will be deleted on sight).
My NIL language has (on the moment I wrote this buggy) support for this. In my current Neil project I am trying to put in this support as well.
In a general sense goes, that only purists (read: people needlessly taking the hard route) will deny the usage of globals is needed sometimes, but in a basic rule goes, use them if you must avoid them if you can.
It's mostly logic thinking, and experience as a coder that can tell you what option is best. Acting like an idiot as soon as you see a global in code is not going to deliver good code. Using globals on places where they can cause more harm than good won't work either. It's just as the tools a carpenter has in his toolbox... if used correctly they deliver a good job... If used incorrectly.... TAKE COVER!!!!
General Blogs: https://trickyjeroenbroks.tumblr.com/
Indie Reviews: https://jeroensindiereviews.tumblr.com/
Game Classics Reviews: https://trickygameclassics.tumblr.com/
0 comments