Back to working on Enemies!
Figured out why they were getting stuck when I changed to them using the Attacking State, turns out I had a true/false check inverted.
Because some Mobs (Mimics) don't animate when asleep I have a check that sets their animation speed 0, but since the check was inverted I was setting Awake mobs image speed to 0, and since the Attack State relies on the Attack Animation ending to exit they were getting stuck.
Also, I was accidentally using the awake variable (used for if things are deactivated) instead of the sleeping variable. Doh.
I've got the Attack system reworked now so damage to the player is done with collision boxes and occurs after the attack animation ends, instead of at the start.
Next I need to work on the attack collisions. I feel like they should only effect the direction the enemy is facing. They should also be centred on the enemy not the player, so that things are more fair and gives you a chance to escape before getting hit.
Of course, some skills like LeechLife can't be avoided, but maybe they should? I could swap them to being AoE skills, with only a single hit allowed.
Changed how the position of the Attack Object is determined.
It now uses the x/y of the player (stored at the start of the Mob's Attack Animation), and the Mob's x/y to get an angle, the feeds that into lengthdir_x and lengthdir_y to know where to create the Attack Object.
I need to adjust the sprites though, when standing below the Mob even though the Player is in range they don't always get hit. This is because of how I'm dealing with the position of the Mobs and their image origin point.
When I was working out the collision boxes I adjusted them all to be offset from the bottom by 4 pixels. This is no longer necessary since I have separate collision and hit boxes..
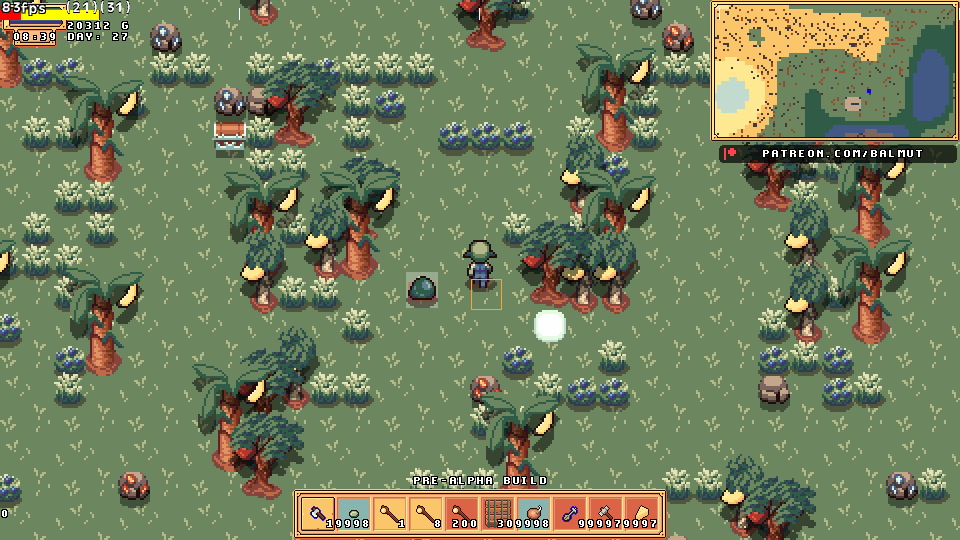
2410
Made that changed to the sprites and also reworked their hitboxes. I wanted to keep them accurate but also fair, as well as being automatic so I don't have to manually set them for every Mob type.
I'm now taking the collision box that's used for movement, expanding that by buffer amount on each side, and then offsetting and cropping by the z amount (z being the distance flying Mobs are from the ground). The box might be a bit big for flying enemies?
(Oh unrelated, but I also added a Slow effect to enemies hit by Ice attacks, which also tints their sprites! Dang I really like the Slime coloured like that...)
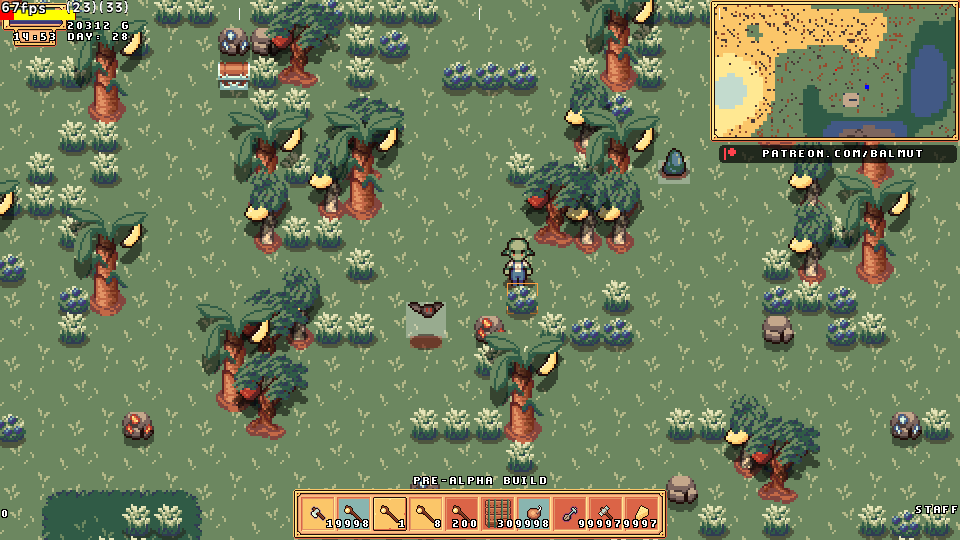
2414
Took a break from Mobs and Skills to do something I've been holding off for ages, swapping the way the world is drawn.
Previously I was just drawing the water then the land, but now I've swapped it.
The main reason for this change is so I can have different colour water depending on location, as it was I had to use different water tiles which was super slow and clunky looking, but now it'll be the land underneath the water that's the different colours and the water shader colour will be applied on top to give everything an extra slight bit of blue tint.
First step is I'm making a temp surface to store the water effect, I'm drawing the texture twice but with different movement directions via shader. Then drawing this same surface over itself with a blue tint (I might be able to skip this and just tint the textures, but this works fine)
Then I'm making another surface with all the areas on screen that water can't be on, and combining this with the water effect shader using a subtract blend so the water effect will only appear on the water tiles. Because the water surface needs to be animated this is later freed up to be made every step.
Next I'm making a permanent surface to store the land, since that only needs to be updated when you Terraform or place paving.
After that it's just drawing them in the correct order.
I haven't tried it yet, but all this should let me make the water wash up and down against the land if I want it to.
https://i.imgur.com/kXpmxGF.gif
2417
It even still works in the Town Menu, I didn't think it did at first but when I was undoing things to try again it did X'D
I still need to figure out a way to do the animated coastline without Objects. Maybe a temp surface will be ok? Gonna try.
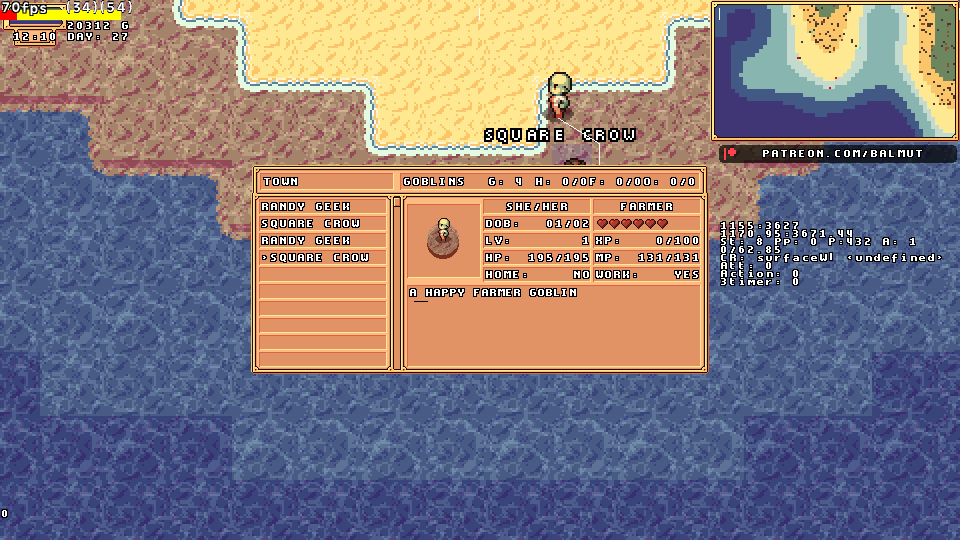
2418
RIGHT! This took quite a bit of messing around, but it seems to all be working now!
Shoreline is no longer done with Objects but entirely with just tiles!
I had to slowly but surely add the animated sprites to the tile sheet for shoreline, which got slower and slower because of all the layers being stored in the image editors memory but eventually it was done (I'm technically short 3 tiles, but unless I decide and figure out how to do rivers I shouldn't need them).
After that I added those tiles and draw conditions to the water surface so that the water would always animate underneath them as it animates, that was the easy part.
Then I had to add them to a new surface so I could actually draw the shoreline, I couldn't just add them to the land surface since that's static and would have to be updated constantly for the animation. So I they got their own temp surface which is made every frame and then that's added on top of the existing land surface. This didn't initially work, but some reordering and code clean up fixed it.
But even though it worked, it was super slow. Turns out my solution of adding things to 2 different surfaces in the same nested for loops is a massive FPS drain, so I swapped to doing a second nested for loop for the shoreline...but then I though why? I can just store the tile data I need in a ds_list during the first for loops and then run through this MUCH shorter list drawing whats needed!
Boom! Perfect! Works in the Town UI too!
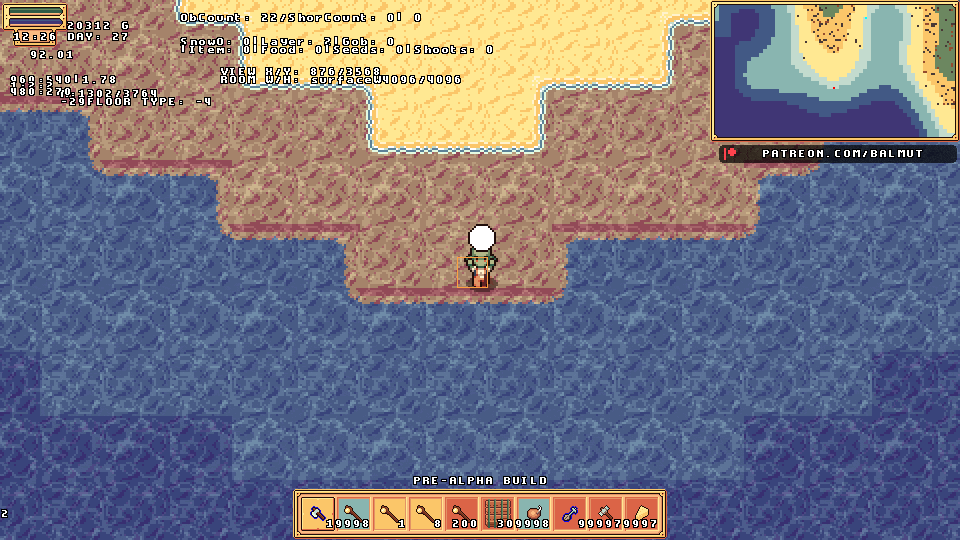
2419
There was still some issues, if a tile contained both shoreline and the edge of the desert only one of them would draw, so I had to do another rework. Eventually figured out the correct order of thing to get it looking right but I had to extend the array that stores the tiles data to be 2x, even if it's neither case.
I thought I was done after that but apparently at some point I removed the GUI check for the top part of water which makes it look deep when touching land. This caused the effect to not happen and for there to be graphical errors since it was working just not offset correctly.
There's only really one thing I want to do with this now, and that's to add a sign wave to the water edges touching land so it's like it's moving, one I work out the shader for it I should be able to just do it as a ds_list like the shoreline.
...I tried to do it and it didn't work. Tried splitting the specific tiles off and applying it only to them, and doing it to the whole water surface and it didn't work. So I'm going to leave it for now.
Next up, update The Underground and The Caves to use the same updated array system as I just made. Gonna be a pain, the code is not localised at all! Mmm spaghetti.
Part way into this update I realised a small issue, and a huge issue
Small issue, I found that destroying walls wouldn't update the floor, it took way to long to realise this was caused by me updating the surface create code into separate surfaces for The Island, The Underground and The Caves. So I added that to the ground surface update.
Big issue, because of the Water Surface, Caves thinner than the view have water visible outside of them. The only way to fix this is to figure out how to crop the view to the room specific (tried and failed multiple times), or resize The Caves to always be at least the view wide. I ended up doing that.
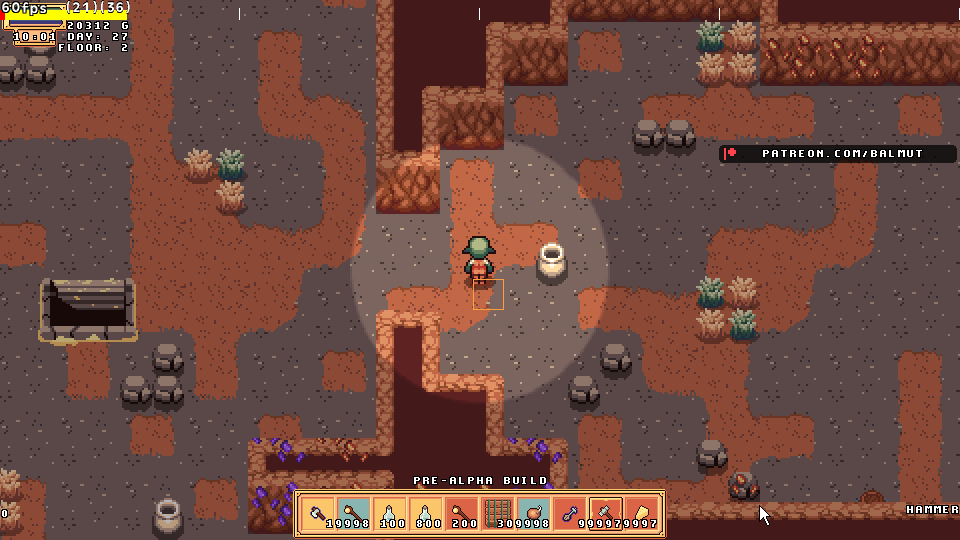
2422
Fortunately with the room size issue I'd previously tried something while working on clamping the view, so I just uncommented that code and started from there. I had to then store the size difference between the cave and the room and apply it to anything being placed so that things wouldn't be placed out of bounds.
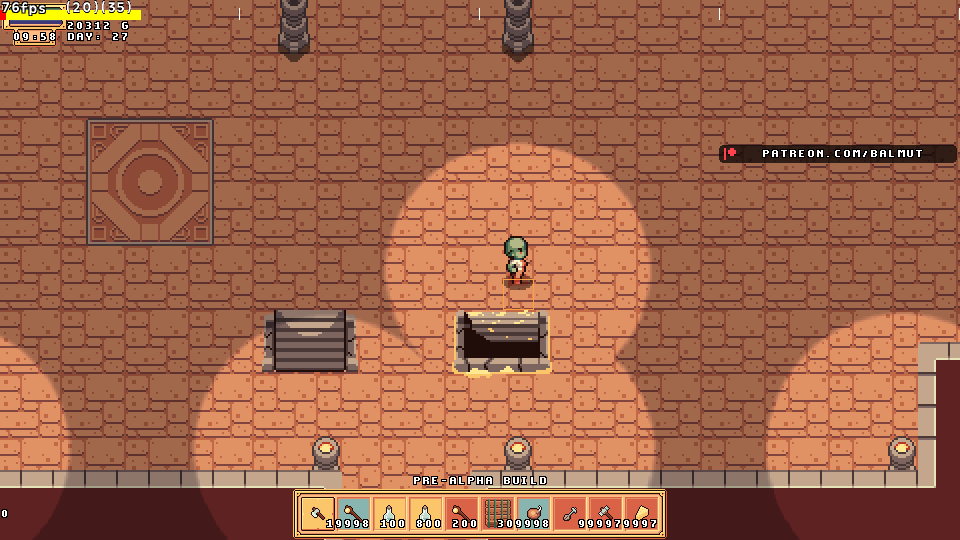
2424
Yay, The Caves and Underground are working again!
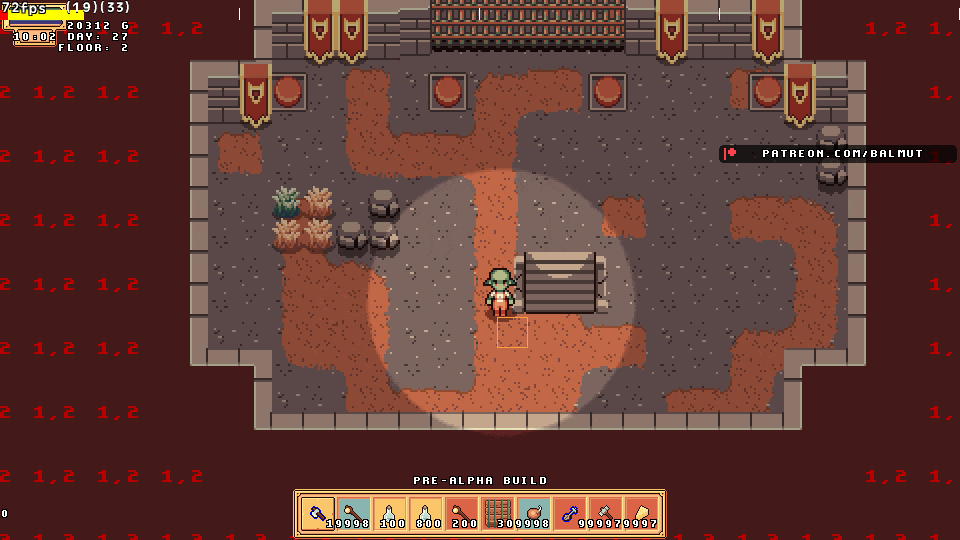
2425
Next a bunch of small updates to how the tiles are dealt with, pretty sure there's currently some redundancy, most can be drawn by the ground draw code, the rest with the surface draw code (stuff that's always over the top), the only things which are needed as actual tiles are the bits that require depth. Like these thin walls, so that you can't see your shadow through them.
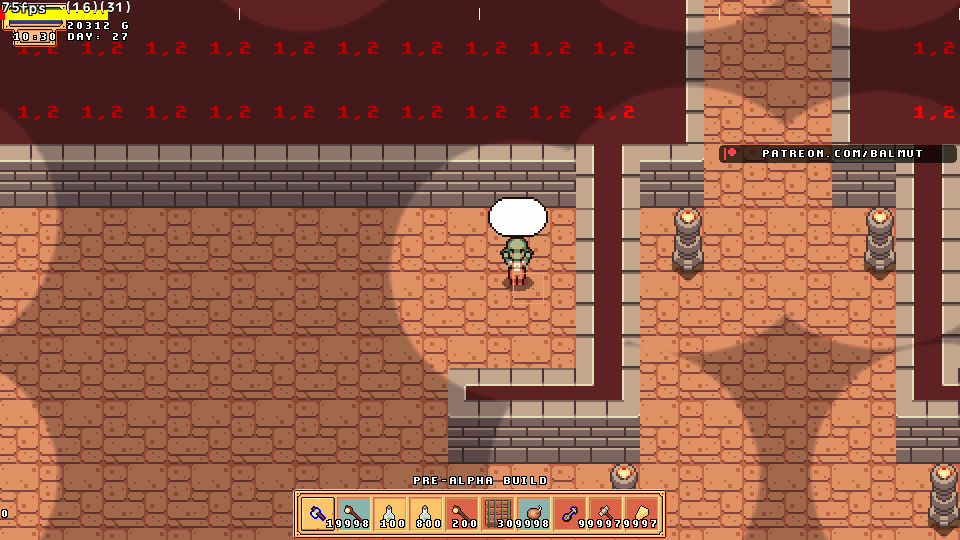
2421
I've noticed that if something occurs to destroy the Islands surface, such as minimising the game window, there is a slight lag spike while it recreates it. I figure this is because it has to recreate the entire surface again. It's barely noticeable, but I'd like to fix it if I can.
I spent a couple days rebuilding things so that I can keep the single nested for loop that currently builds the water surface for the water that's in view, and store the land in a ds_list to be built to it's own surface. Unfortunately my early attempts turned out to be way slower and I ended up with issues (I'm assuming due to draw order) where some Beach tiles at the shoreline would have the wrong colour sand below them. It only happens to the corner ones, not the flat parts?!
Two days later and I've given up on this, I still have the code so I can keep trying at a later time.
Next I tried saving the Islands surface as a background, figuring that would be quicker to just reload that instead of having to loop through the grid every time but I didn't notice any difference. It's only a small change so I've commented it out to revisit later.
Tried a new method of generating Ore Veins but it didn't really work, I wanted to use the same system that I use for generating the island, at first I couldn't get it to output anything. I did a full rewrite but couldn't get it to output anything decent. I'll try again in the future.
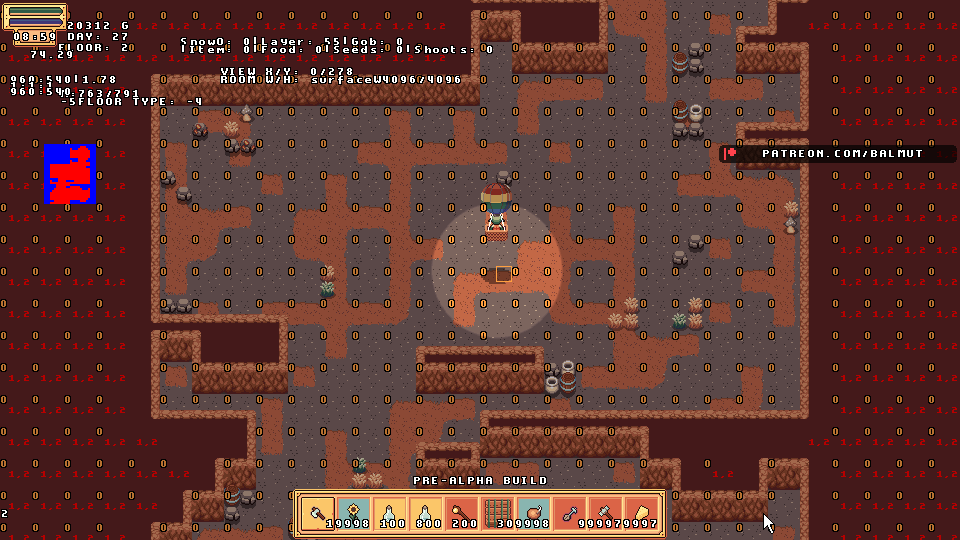
2426
Added Culling to The Underground, since I've been populating it with new stuff. Slight issue that now there is pop-in and because the stairs are culled I can't spawn next to them. Quick fixes:
save the stair coords as a variable.
reorder the code, so that camera is placed at the player immediately after spawning, so the stairs can be unculled, then move the player to them.
Oh hey! New Underground Torches! No I don't have to worry about the 1x1's not being aligned to the tile grid!
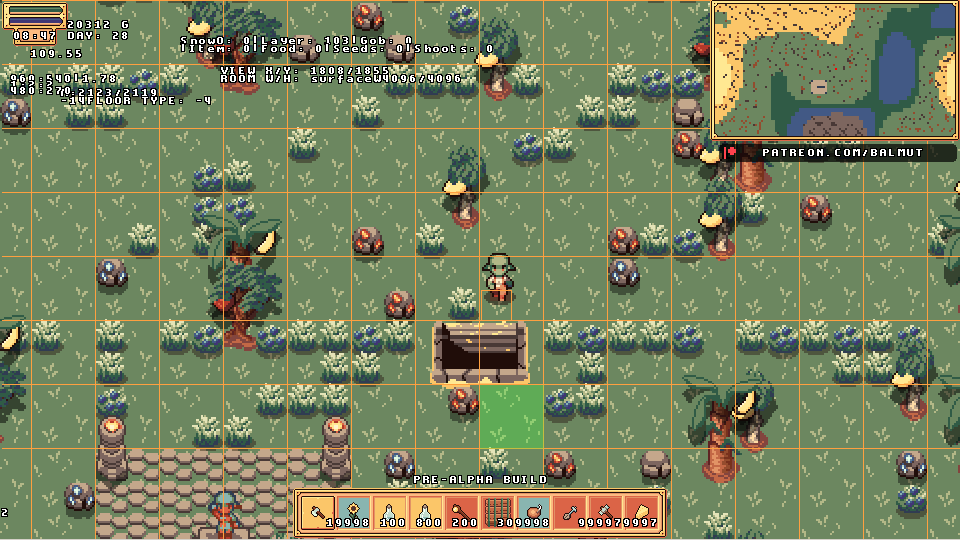
2427
New Underground Doors! Version 1.
https://i.imgur.com/z4KNKSb.gif
2428
Also in Vertical orientation! Slight issue that Goblins can walk through part of them. This is because their Pathfinding uses the sub-tiles not the collision box of objects, so I'm going to have to redesign them. I think the best option is to make the doors retract completely into the wall. Also, another new Underground light, Wall Torches!
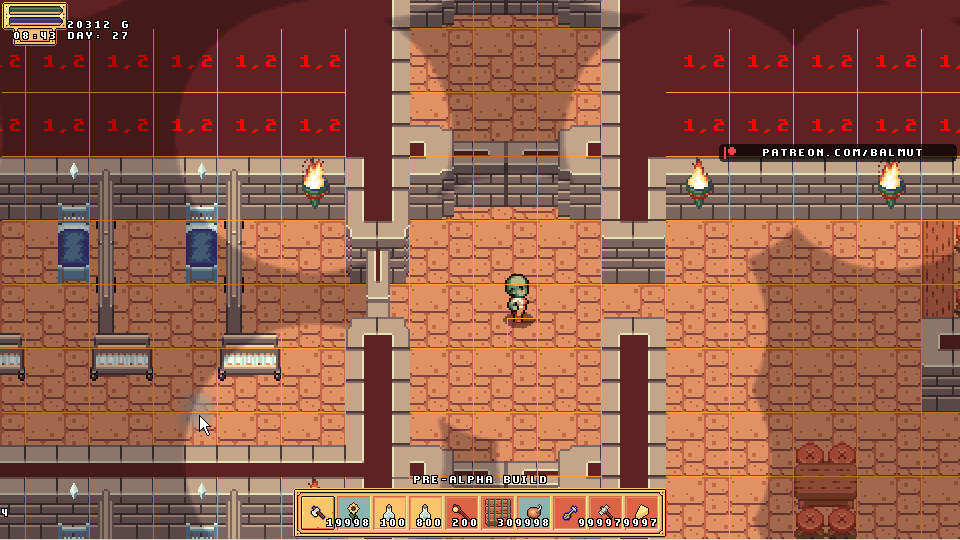
2429
Underground Doors Version 2, now receding completely flush!
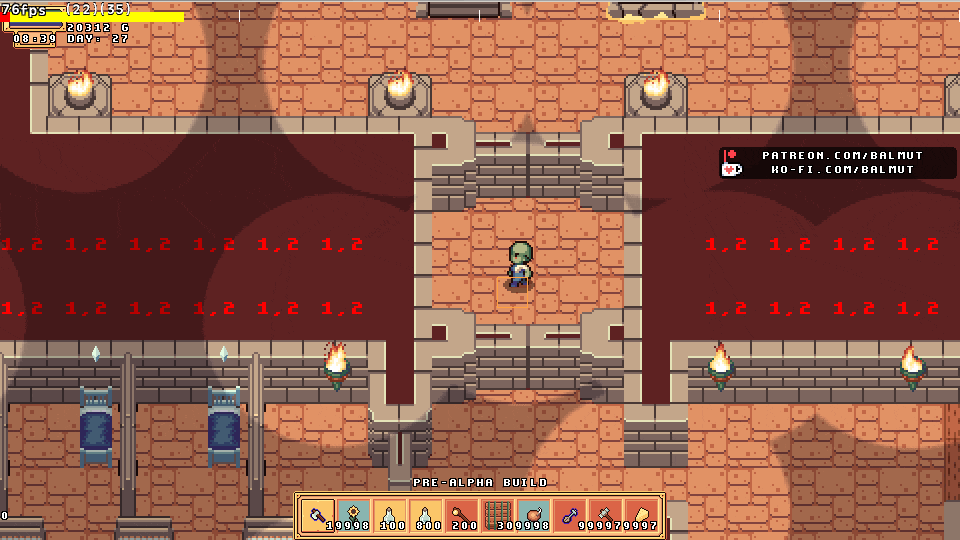
2435
It wasn't much, but for April fools I changed some settings and made the Goblins into Aliens. X'D
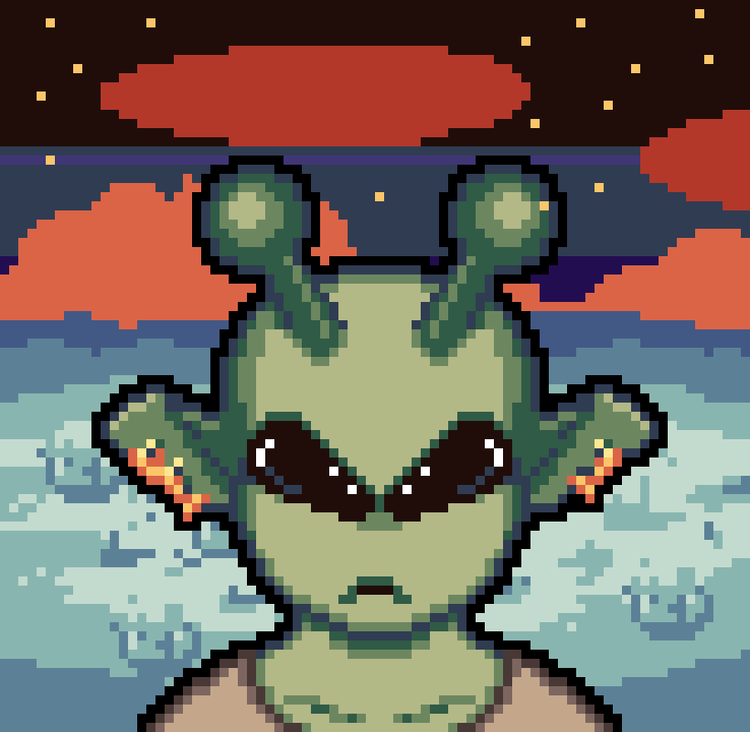
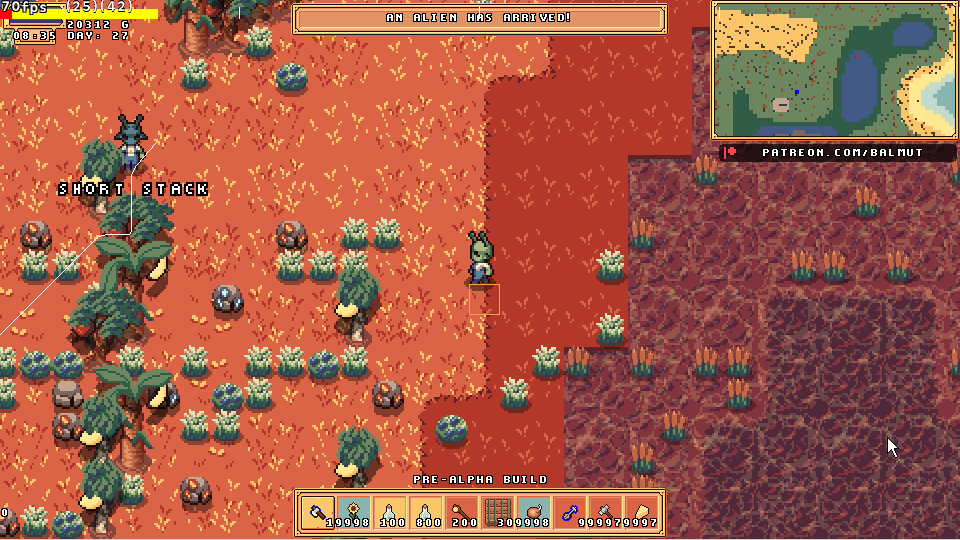
2430
In preparation for adding the first non-random NPC I've
Added new face shapes.
Added new hats.
Added new clothes, based on jobs.
Reworked how faces are generated.
Rebuilt how they are drawn, to allow clothes be be under their chin while talking.
Since some parts conflict with each other, I've made them not possible to be generated together.
There was a slight issue with the cropping on hats and clothes, since they can be bigger than the portraits they've been cropped on the spritesheet, need to adjust the size of it before importing again. (Fixed)
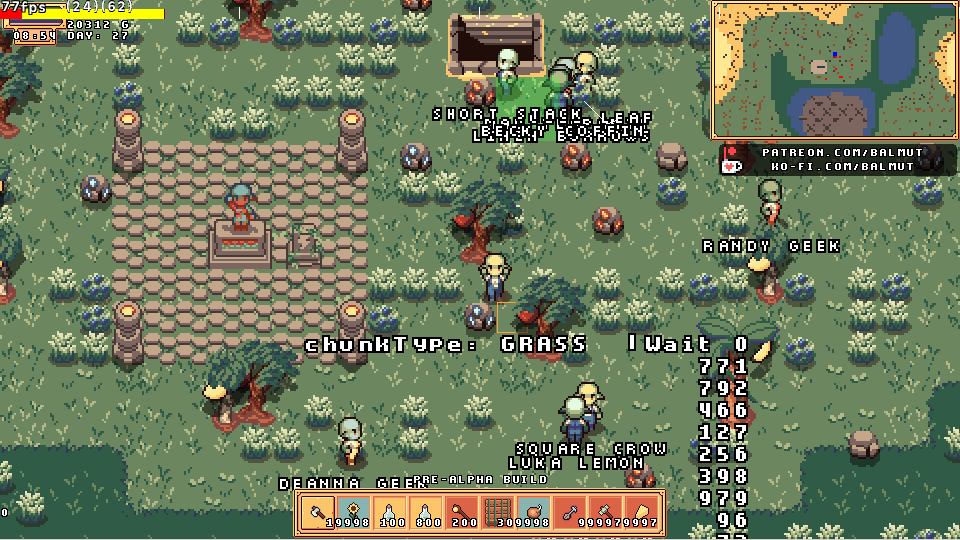
2433
Bug Fixes and small Changes
Fixed a bug making World Spawned Plants causing a crash.
Fixed The Caves minimap shape.
Fixed depth of Plants in Plant Pots.
Fixed bug where Underground Garden would fail to generate plants and cause a crash.
Fixed explosions from Exploding Walls not being centred.
Fixed NPC's getting stuck in walls.
Fixed NPC's not being able to leave chairs in certain directions.
Fixed ore veins in The Caves being RNG on every tile, instead of each vein.
Fixed a BUG (totally not me doing it with a button) that caused The Cave exit stairs to disappear.
Fixed Object pop-in when going to The Underground.
Changed the draw order of things done by the Surface Controller, so that the tops of Walls are drawn before the Lighting surface.
NPC's can now correctly find the stairs to return to the Island from The Underground.
And I think that's everything for the month?
Thank you all for your support it truly means the world to me.
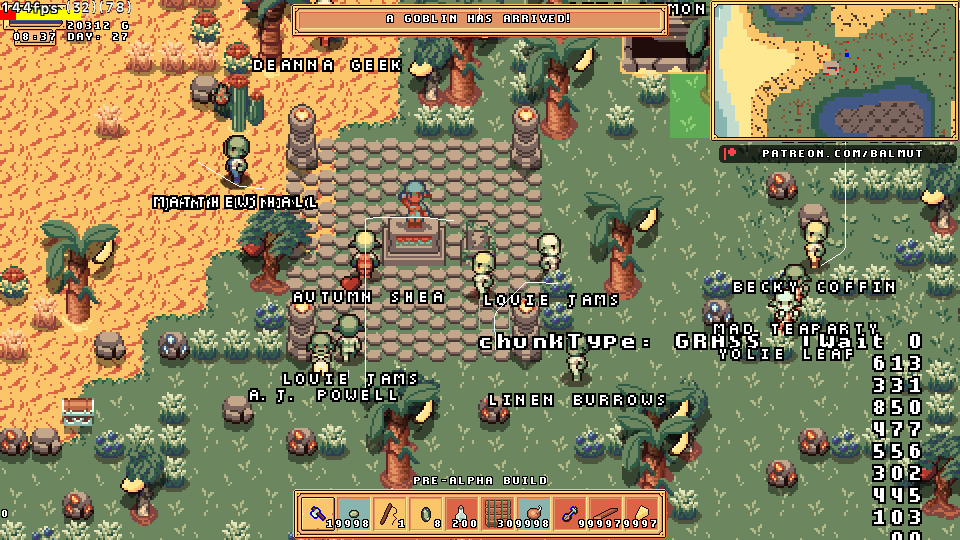
Please stay safe out there, look after yourselves and keep being awesome.
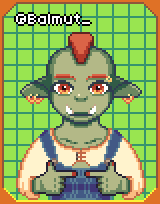
1 comment